Trying to find a file containing a certain string of text using PowerShell? Well, you’ve come to the right place. I’ll show you how to find exactly what you’re looking for with the Select-String
cmdlet and a basic PowerShell script.
How to use the Select-String PowerShell cmdlet
The Select-String
cmdlet is a PowerShell command used to identify text patterns. Select-String
is commonly used to identify text strings in files, but it can be used to identify strings from other sources as well.
Here’s a simple example of how to use the Select-String
cmdlet.
Get-Command | Select-String -Pattern 'Appx' -SimpleMatch
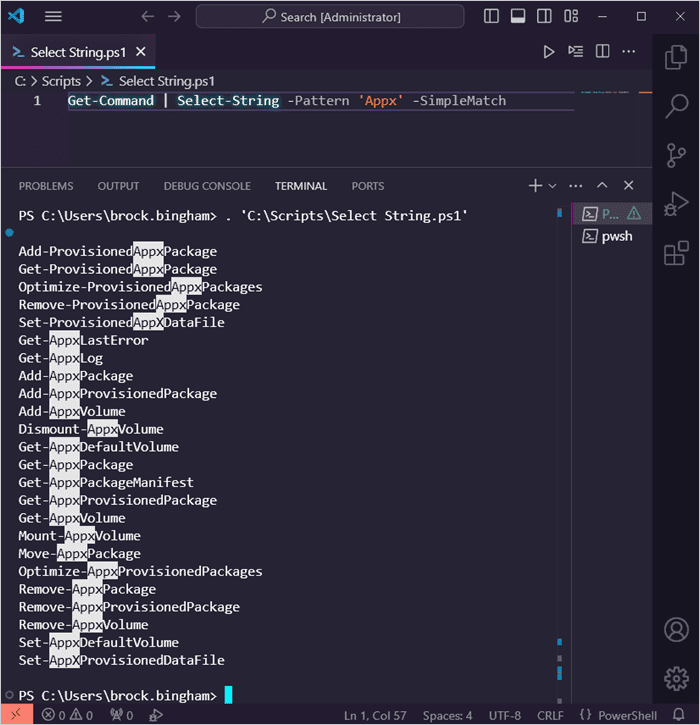
In this example, we pipe the results of Get-Command
to the Select-String
cmdlet and filter with the pattern ‘Appx.’ We add the -SimpleMatch
parameter so the command doesn’t interpret the pattern as regular expression, which Select-String
does by default. In the end, this example returns all the PowerShell commands that have the string ‘Appx’ in the name.
How to identify a text string in a file with PowerShell
As I mentioned in the previous section, one of the primary uses of the Select-String
command is to search for text strings in a file. Let’s start with an easy example to get our feet wet.
Select-String -Path 'C:\temp\*.csv' -Pattern 'Bart.Simpson' -SimpleMatch
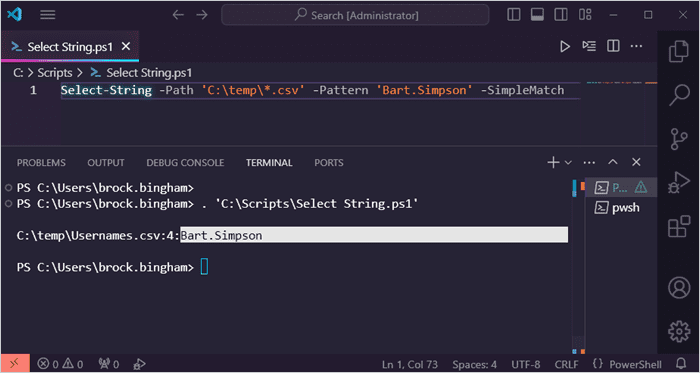
In this example, we’re searching files for the string pattern ‘Bart.Simpson.’ For the path, we’re searching the C:\temp directory, specifically looking for .csv files. As you can see in the screenshot, we found a matching result on line 4 of a file called Usernames.csv.
If there are multiple sources found that match the pattern, the command returns each source. To show this off, I’ll run the command again, but this time I added a second file containing the pattern we’re looking for.
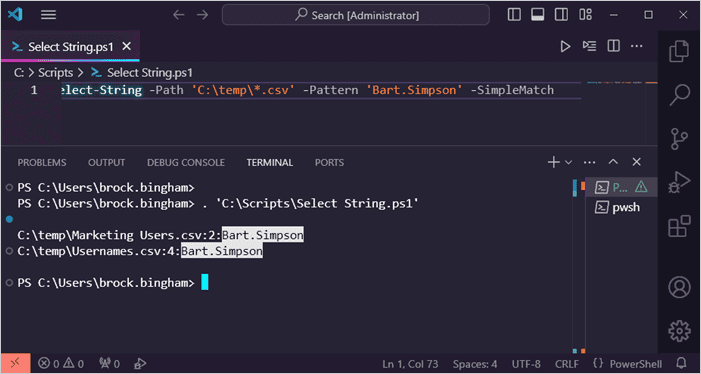
The command now returns both sources where the string was found.
How to search subdirectories for a string with PowerShell
If you’re familiar with PowerShell, you’ve probably used the -Recurse
parameter to search subdirectories. However, the Select-String
command doesn’t support the -Recurse
parameter. That’s okay, though, because we can use Get-ChildItem
with the -Recurse
parameter and then pipe the results to the Select-String
command. Here’s an example.
Get-ChildItem -Path 'C:\temp\*.csv' -Recurse | Select-String -Pattern 'Bart.Simpson' -SimpleMatch
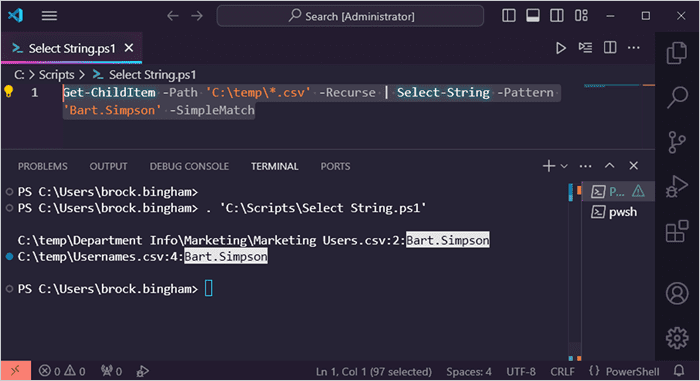
In this example, we use the Get-ChildItem
command to define the directory path and use the -Recurse
parameter to ensure that subdirectories are included. The results are then piped to the Select-String
command. We don’t have to redefine the path since it was already set in the previous command.
This example returned two results, one in the C:\temp\ directory and the other in the C:\temp\Department Info\Marketing\ subdirectory.
How to find a date with Select-String and regular expression in PowerShell
Sometimes you don’t know the exact data you’re looking for. You might only know the format of the data you’re trying to find. That’s when regular expression (regex) comes in handy.
When most people have nightmares, they’re usually about snakes, bad guys, or not being properly clothed in a public setting. When I have nightmares, they’re usually about regex. Thankfully, there are a ton of great regex resources on the internet, including our very own PowerShell regex guide. Make sure to check it out if you want to learn more about regex and how to use it with PowerShell.
Here’s an example that uses Select-String
and regex to identify strings that match a MM/DD/YYYY format.
#Set the regex pattern to identify MM/DD/YYYY format and set it to a variable
$dateRegex = '(0[1-9]|1[012])[- /.](0[1-9]|[12][0-9]|3[01])[- /.](19|20)[0-9]{2}'
Select-String -Path 'C:\temp\*.csv' -Pattern $dateRegex
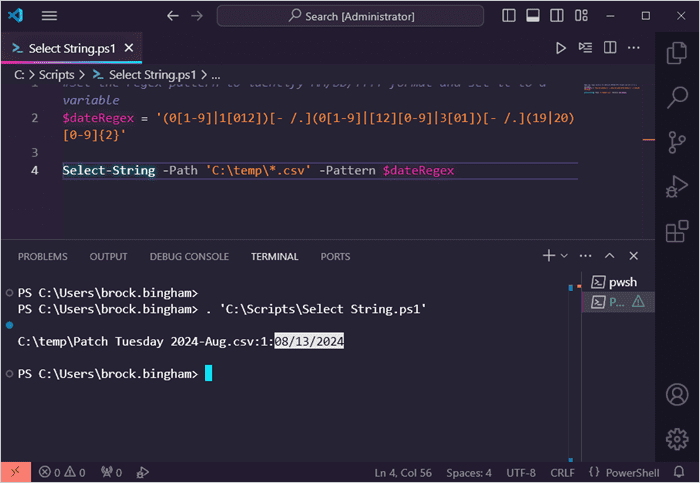
The results returned a matching date format in line 1 of a file at “C:\temp\Patch Tuesday 2024-Aug.csv.” The returned string was the date 08/13/2024. Notice that we didn’t use the -SimpleMatch parameter to ensure the regex was properly read.
How to find strings in Windows event logs with Select-String
Another beneficial use case for the Select-String
cmdlet is to identify specific strings in Windows event logs. This one is great for anyone that really doesn’t like browsing through Event Viewer to view logs.
In this example, I use the Get-WinEvent cmdlet because I’m using PowerShell 7.x. If you’re using Windows PowerShell, you’ll want to use the Get-EventLog command. Or, you know, you could join the rest of us and use PowerShell 7.x. Andrew Pla, host of the popular PowerShell Podcast, would be so proud of you.
Here’s an example of how to search for a string in Windows event logs with the Select-String
cmdlet.
$logs = Get-WinEvent -LogName Setup -MaxEvents 50
$logs | Select-String -InputObject {$_.Message} -Pattern 'KB5044020' -SimpleMatch
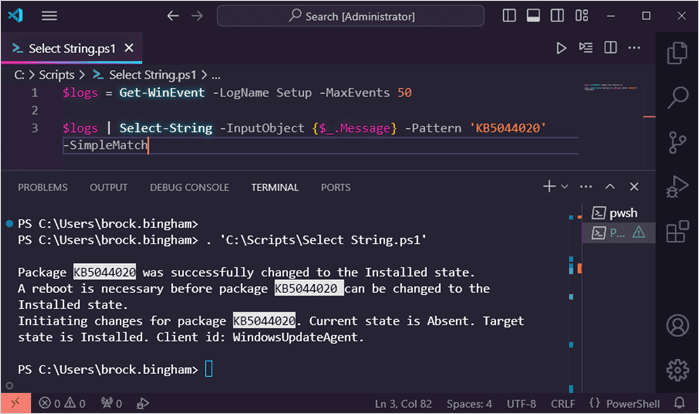
In this example we’re returning the 50 most recent event logs from the Setup logs and assigning it to the $logs
parameter. We then pipe the results to the Select-String
command, specifically searching the log messages for the pattern KB5044020. Lastly, we use the -SimpleMatch
parameter to ensure the pattern is read as text and not a regular expression.
How do YOU use the Select-String command?
These examples should help you get started using the Select-String command. But I want to hear how you use the Select-String command in your scripts. Join the PDQ Discord server and let me know.